Monday, June 15, 2009
Is Mathematics Important?
Mathematics is one of the oldest professions, and it has been maintained and extended by some of the greatest minds in human history. Like many sciences, mathematics gave birth to computer science, and it is used by computer scientist to solve problems and to convey research formally. Mathematics is the beating heart of computer science, and it is the most important language for a programmer to know.
Although programmers can reference highly polished algorithms from books, they can have trouble determining where to use them if they do not have a mathematical background. Mathematics provides the tools that programmers need to measure the efficiency and usefulness of algorithms. Since every algorithm has advantages and disadvantages, it is very important for programmers to select the appropriate algorithm to solve a problem. If programmers select poor algorithms for problems, they will develop slower and less efficient software even though they may be using a lower level language. Programmers need to be able to measure and judge the efficiency of algorithms so that they can write high performance software.
In addition to performance, mathematics eduction teaches programmers essential problem solving skills that are useful for programming. Programmers can translate and use many of the formulas of mathematics in their code. For example, programmers can use graph theory to design special data structures or messaging systems for use in their software. Programmers also learn many mathematical rules that they can apply to aid in solving problems. Programmers with knowledge of mathematics are better programmers because they have essential problem solving skills and access to a large array of algorithms.
Besides the educational benefits, mathematics contains powerful tools that programmers can use to check their algorithms for safety and quality. Programmers can use mathematics to verify the design of their code so that they can have mathematical guarantees for security. Programmers can also use mathematics to rigorously prove their code works so that they do not have surprising errors after they release their software. Since society is becoming more dependent on software, programmers will be under increasing pressure to produce secure and reliable code, and mathematical knowledge is essential for programmers to accomplish writing rigorous code.
In Conclusion, programmers should learn and practice mathematics so that they can write more secure, reliable, and fast applications. Programmers who master mathematics can better test their algorithms for security issues, and they can better test their software for quality. Programmers can also use mathematical analysis on their algorithms so that they can produce faster code. In addition, programmers learn essential problem solving skills from mathematics that they need to solve new problems. Mathematics is the heart of computer science, and programmers need to know it to better their profession.
Read More......
Friday, June 12, 2009
Single Linked List in Visual Basic.Net
Linked Lists are created out of user defined types called nodes. A node is a class or data structure that is used to store a group of information. For example, a node may contain information about customers like their first names, last names, and social security numbers. In a single linked list, a node will also contain a special variable that points to the next node if one exists. When a new node is created, the last node of the listed will update its special variable to point to the new node. Since only one variable is used in a single linked list, it can only be traveled in one direction from head to tail. The following figure is a diagram of how a single linked list is structured.
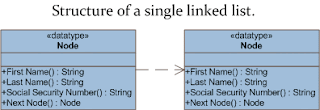
In visual basic, nodes can be created with classes. The following code is an example of how to create a class node. The node is going to be used to store some example information of a customer.
'Example of a node for use in a link list
Public Class clsNode
Private m_FirstName As String 'First Name of customer.
Private m_LastName As String 'Last name of customer.
Private m_SSN As String 'Social Security Number.
Private m_next As clsNode 'Pointer to next node
Sub New()
m_next = Nothing 'Default to nothing
End Sub
Public Property FirstName() As String
Get
FirstName = m_FirstNameEnd Get
Set(ByVal StrName As String)
m_FirstName = StrNameEnd Set
End Property
Public Property LastName() As String
Get
LastName = m_LastNameEnd Get
Set(ByVal strLastName As String)
m_LastName = strLastNameEnd Set
End Property
Public Property SSN() As String
Get
SSN = m_SSNEnd Get
Set(ByVal strSSN As String)
m_SSN = strSSNEnd Set
End Property
Public Property NextNode() As clsNode
Get
NextNode = m_nextEnd Get
Set(ByVal clsNextNode As clsNode)
m_next = clsNextNodeEnd Set
End Property
End Class
Now that the node class is created, lets move on to creating a linked list class. The following code is an example of how to create a single linked list. The example linked list is just going to have simple functionality, and programmers should add more to it in order to better understand linked list data structures. A method to insert a node into a specified position of the list, remove a single node from any position in the list, and the ability to sort the list would be interesting functions for programmers to add.
Public Class clsSingleList
'Member Data
Private m_Head As clsNode 'Stores the first node.
Private m_Tail As clsNode 'Stores the last node
Private m_count As Long 'Count of nodes
'Funciton New - Default Constructor
'Called when class is initialized.
Sub New()
m_count = 0 'The list has no nodes.
m_Head = Nothing 'Set head and tail to nothing.
m_Tail = Nothing
End Sub
'Function InsertAtHead
'Purpose: Clients should call this function to insert
'a node at the head of the list.
'Input: Node to be inserted.
'Output: none
'--------------------------------------------------------------------
Public Sub InsertAtHead(ByVal vNode As clsNode)
'If head is set to data.
If Not IsNothing(m_Head) Then
'The new node should be linked to head.
vNode.NextNode = m_Head'set head as new node.
m_Head = vNode
Else
'First node in the list.
m_Head = vNode
m_Tail = vNode
End If
'update count
m_count = m_count + 1
End Sub
'Function InsertAtTail
'Purpose: Clients should call this function to insert
'a node at the tail of the list.
'Input: Node to be inserted.
'Output: none
'------------------------------------------------------
Public Sub InsertAtTail(ByVal vNode As clsNode)
'if head is set to data
If Not IsNothing(m_Head) Then
'Link last tail to new node
m_Tail.NextNode = vNode'Update tail to the new node.
m_Tail = vNode
Else
'Only node in the list.
m_Head = vNode
m_Tail = vNode
End If
'update count
m_count = m_count + 1
End Sub
'Function GetNode
'Purpose: Clients should call this function to retrive
' a node in the list.
'Input: Position of node in the list.
'Output: The node in the requested position. If no node
' exists, the function will set the clsNode to nothing.
'------------------------------------------------------
Public Function GetNode(ByVal index As Integer) As clsNode
Dim cur As clsNode 'Temp var used to walk list.
Dim i As Integer = 1 'Temp Counter'If client requested a position in the list that
'does not exist, set the return node to nothing,
'and exit funciton.
If index > m_count Then
GetNode = Nothing
Exit FunctionEnd If
'Start at the top of the list.
cur = m_Head'Walk the list until the desired position is located.
Do While i <>
cur = cur.NextNode
i = i + 1Loop
'Return the node
GetNode = cur
End Function
'Function: Count
'Purpose: Clients should call this function to retrive
'the number of nodes in the linked list.
'Input: none
'Output: Number of nodes in list.
'------------------------------------------------------
Public Function Count() As Long
'Return Count
Count = m_count
End Function
'Function Empty
'Purpose: Clients should call this function to remove
'all nodes in the list.
'Input: none
'Output: none
'------------------------------------------------------
Public Sub Empty()
m_Tail = Nothing
m_count = 0
'Walk through list to remove all references to
'node objects. The garbage collector will take
'care of the actual freeing for us after it sees
'no references to the nodes.While IsNothing(m_Head) = False
m_Head = m_Head.NextNodeEnd While
End Sub
End Class
Finally, the following is an example of how to work with the linked list.
Private Sub Button1_Click(ByVal sender As System.Object, ByVal e As System.EventArgs) Handles Button1.Click
Dim i As Integer
Dim mynode As clsNode
Dim mylist As New clsSingleList 'Our list
'Create a new node.
mynode = New clsNode
'Give it some data.
mynode.FirstName = "John"
mynode.LastName = "Jones"
mynode.SSN = "555-55-5555"
'Add it to the front of the list.
mylist.InsertAtHead(mynode)
'Create a new node
mynode = New clsNode
'Give it some data
mynode.FirstName = "John"
mynode.LastName = "Homes"
mynode.SSN = "555-55-5555"
'Add it to the tail of the list
mylist.InsertAtTail(mynode)
'Print Count
Debug.Print(mylist.Count)
'Walk list
For i = 1 To mylist.Count
mynode = mylist.GetNode(i)
Debug.Print(mynode.FirstName)
Debug.Print(mynode.LastName)
Debug.Print(mynode.SSN)
Next
' Empty List
mylist.Empty()
Debug.Print(mylist.Count)
End Sub
In conclusion, linked list data structures are easy to create in visual basic. Since linked lists work on generic data, visual basic programmers can reuse their linked list code in many programs. After programmers build up a collection of different data structures, they can use them to solve many complex problems.
Read More......
Thursday, June 11, 2009
Is Visual Basic a serious language?
Software development has grown into a complex process. In the past, one or two programmers could create and maintain world leading software; however, modern day software requires large development teams and interdisciplinary people. As software development increases in complexity, the cost of software development increases as well. Programmers need powerful tools to deal with the ever increasing complexity of software; indeed, they need tools like Visual Basic.
While jaded programmers doubt the power of Visual Basic, they understand that software development is rapidly growing in complexity. Visual Basic provides abstractions to help simplify problems, and it provides a fast turn around time for development; therefore, the cost of development in Visual Basic is considerably smaller in comparison to many other languages. Visual Basic also leaves behind the baggage found in many other languages. Memory leaks, runaway pointers, and buffer overflows are not as large of a problem in Visual Basic as they are in other languages because Visual Basic manages details that are error prone. Even though some programmers doubt the power of Visual Basic, they can see some of its advantages.
The Visual Basic language allows programmers to use object oriented programming, construct logical statements, and implement important data structures. Object oriented programming is a very powerful feature because it allows programmers to build up libraries of reliable code. Programmers can use object oriented programming to continually add layers to complex problems so that the problems become simplified and easier to work with. In addition to OOP., Visual Basic allows logical statements like shift, and, or, and xor that are fundamental to powerful programming. Programmers can also implement data structures in Visual Basic like link lists, stacks, queues, and trees. Visual Basic has all the important features that are found in other languages while it leaves much of the error prone baggage behind.
Although visual basic is a great language, some programmers disagree. Some programmers believe that Visual Basic lacks powerful features, but Visual Basic programmers can implement any data structure that can be implemented in C++. Since Visual Basic allows programmers to solve problems rapidly, some programmers believe that it must effect the software quality; however, it increases the software quality because programmers are working with complex problems through layers of abstractions. Many programmers believe that Visual Basic is too slow to be useful, but it is often as fast as comparable software written in other languages. While languages such as C++ are useful in special areas of programming, Visual Basic adds much to a programmers tool-belt.
A small amount of programmers believe that Visual Basic encourages bad programming practices and is used by stupid people, but they are misguided. Visual Basic is a great language for first time programmers, and it has a larger number of new programmers who are learning the basics of programming; however, knowledgeable visual basic programmers follow good programming practices. Like Visual Basic, poor programming practices are prevalent in other languages as well. The battle between security experts and hackers is intense due to wide spread use of poor programming practices in all languages. Hackers have no trouble finding countless exploits in C and C++ applications because of poor programming practices and lazy programmers; also, users have no trouble finding run away pointers and memory leaks. In a basic nutshell, a formal education in computer science and mathematics is what separates good programmers from bad programmers.
In conclusion, Visual Basic is a powerful tool in the hands of knowledgeable programmers. Since computer hardware is moving to multiple core processors, software is going to become increasingly difficult to program. Computers are also prevailing in unlikely places such as phones, cars, and other personal gadgets, and they are all interconnected into a vast network called the internet. Programmers need powerful tools that provide abstraction, and Visual Basic is a very powerful tool for programmers to add to their toolbox.
Read More......
Generating hash values for strings (VB.net)
For programmers who want to understand how hashing works, I'm going to explain the process of a very weak hashing algorithm. A rudimentary hash function can be created by summing the ASCII (American Standard Code For Information Interchange) values of every byte of data in a string. For example, the character 'A' has an ASCII value of 65, and the character '5' has the ASCII value of 53. The hash value of the string “A5” would be 65+53 = 118. If the string is changed to “A4”, the hash value is changed to 65+52 = 117; therefore, we would be able to detect the modification of our data.
Note: Individual characters can be displayed in visual basic with the Asc function or obtained in the character map in the start menu. Example: MsgBox(Asc("A"))
While our rudimentary hash function works, it has one major problem. What happens if someone changes the string to “K+”? Our hashing function would generate the value 75 + 43 = 118! This problem is referred to as a collision (two different strings that produce the same hash value), and our simple hashing example has many collisions. Unlike our example, the hash functions provided by the .Net library are mathematically construct to have very few possible collisions.
The .Net framework provides several different hash functions that visual basic programmers can implement into their applications. Like encryption, Greater bit sizes usually correspond to greater protection. The following is a list of the different hash functions and hash value sizes that are defined in the namespace Security.Cryptography.
SHA-1, Generates 160 bit hash values.
SHA-256, Generates 256 bit hash values.
SHA-384, Generates a 384 bit hash value.
SHA-512, Generates a 512 bit hash value.
MD5, Generates 128 bit hash values.
Finally, the following function illustrates how visual basic programmers can use the hash functions provided by the .Net framework. The function accepts string input and outputs the hash value of it.
'''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''
'Function GenerateHash
'Purpose: Generate a hash value for string data.
'Input: strbuffer, a string varable of text to be hashed.
'Output: The hash value in a base64 encoded string.
'''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''''
Private Function GenerateHash(ByRef strbuffer As String) As String
'Encoder is used to convert strings into btyes and vise versa
Dim myEncoder As New System.Text.UnicodeEncoding
'The hash function expects the input to be in bytes.
Dim myBuffer() As Byte = myEncoder.GetBytes(strbuffer)
'Here we dimension our MD5 hash function.
'Uncomment other algorithms to try them out.
''''''''''''''''''''''''''''''''''''''''''''''''''''''''''
Dim myhash As New Security.Cryptography.SHA512Managed
'Dim myHash As New Security.Cryptography.MD5CryptoServiceProvider
'Dim myHash As New Security.Cryptography.SHA1Managed
'Dim myHash As New Security.Cryptography.SHA256Managed
'Dim myhash As New Security.Cryptography.SHA384Managed
'The following line does a few different things.
'1. It generates a hash value and returns it in bytes.
'2. The encoder is used to convert the bytes into a base
' 64 string.
'3. The function returns the string value.
GenerateHash = Convert.ToBase64String(myHash.ComputeHash(myBuffer))
End Function
When information needs to be checked for modification, it should be hashed, and the new and old hash values should be compared.
Read More......
Wednesday, June 10, 2009
Optimization: Avoid Redundancy
Redundant code inside of loops can be very taxing on software performance. One unnecessary instruction inside of a loop can have a large impact on the performance of software because it is repeated with every iteration of the loop; therefore, programmers should carefully check loops for unnecessary instructions. Visual basic programmers should also avoid accessing the properties of objects inside of a loop; instead, they should cache the needed properties inside of variables. In addition to properties, programmers should avoid function calls inside of loops because function calls are expensive operations. Loops are the most critical part of software, and programmers should pay careful attention to avoid redundant code in them.
For example, the following code has an instruction that never changes; as a result, the software will repeat the useless instruction fifty times.
for i = 1 to 50x = b ' Stays the same with every loop, get it outside of the loop!next i
k = j + I
j = j + 1
The above code should be replaced with the following to avoid redundancy.
x = b 'Moved outside the loop
for i = 1 to 50
k = j + I
j = j + 1
next i
In another example, the following code accesses a property inside of a loop. Every single dot corresponds to one function call.
for i = 1 to 50
text1.text = text1.text + b(i) '100 function calls.
next i
In the above example, two function calls are made with every iteration of the loop. To avoid the function calls, the data can be cached in a variable then assigned to the property after the loop is finished.
for i = 1 to 50
strbuffer = strbuffer + b(i) ' No function calls.
next i
text1.text = strbuffer
Finally, the following example illustrates a redundant function call. Many visual basic programmers use built in functions carelessly inside of loops, but it should be avoided for performance reasons.
for i = 1 to 100
sz = len(string) ' One hundred function calls
'Do processing
next i
The above code can be simplified to the following correction.
sz = len(string) ' One function call
for i = 1 to 100
'Do Processing with sz
next i
Programmers should always search for unnecessary calculations and poorly designed decision nests in their code. Unnecessary calculations are a frequent problem in code because many programmers are not mathematically strong. Many programmers include additional instructions to acquire a result that can be obtained with fewer calculations. Aside from calculations, programmers should be careful when they design decision nests in code. The conditional branch that is most likely to be true should be placed the highest in a decision nest, and the conditional branch that is most likely to be false should be placed the lowest in the nest. If programmers pay careful attention, they can avoid unnecessary calculations and design better decision branches.
In the following example, the calculations being made are redundant because parts of the calculation can be shared. The variables a and b are multiplied twice.
x = a * b + c
y = a * b + d
To avoid redundancy, programmers can simply share parts of the calculations.
t = a * b
x = t + c
y = t + d
Programmers should avoid redundant memory allocation as much as possible. Memory allocation is a very expensive process to perform in code, and it is frequently a topic in optimization. Like hard drives, frequent memory allocation and freeing of small data may cause memory fragmentation. After computer memory becomes fragmented, computer performance is severely effected because the memory manger must find appropriate memory locations that are large enough to store data. When programmers need to allocate memory, they should attempt to allocate enough memory to avoid the need for reallocation.
In conclusion, visual basic programmers can speed up their applications by reducing code redundancy. Code redundancy is a common occurrence in visual basic programs, and it can be avoided with careful attention to detail.
Read More......
Introduction to Optimization
In many situations, code optimization can often be avoided by using good programming practices. Writing code is much like writing arguments or essays, and good programmers will often write an outline of the software program before they sit down to code it. Outlines allow programmers to focus on the clarity of the software without having to worry as much about details. After an outline is created, programmers should evaluate individual functions for algorithm selection. Since algorithms have strengths and weaknesses, programmers should select the right one for the task at hand. Like in English, programmers should also proof read for errors and redundancy. Good programming practices can reduce development time and provide better quality software, and they can also lead to more responsive software.
Many programmers often waste time optimizing irrelevant code. Optimizing every single line of a program is poor programing practice and a complete waste of time. When a program has speed problems, it should be profiled to identify areas in code that are causing the latency. Loops and complex calculating instructions should be the targeted areas during the optimization process. When an algorithm is identified as being the cause of performance loss, the algorithm should be evaluated by big-O notation before optimization is considered. If an algorithm has a poor big-o, it would be a waste of time to optimize the algorithm. Programmers must be able to identify areas in code that cause performance problems so that they do not waste time optimizing useless code.
Optimized code can be very difficult to maintain. Documentation is very important when code is being optimized because optimized code can be cryptic to read. Since optimized code is so complex, programmers should always provide the algorithm being used before optimization in their documentation. Aside from providing the unoptimized algorithm, programmers should detail every step of the optimization process so that they can understand the algorithm at a later date. Documentation is a critical part of the optimization process, and good programmers will always document optimized code rigorously.
In conclusion, programmers must follow good programming practices as they become increasingly responsible for performance gains in the computer industry. Programmers should always follow the software development cycle and proof read their code. During the coding process, programmers should rigorously document optimized code to avoid errors and maintenance nightmares.
Read More......
Passing Visual Basic Arrays to Assembly DLL Modules
While a complete introduction to assembly language is beyond the scope of this article, programmers who know some of the x86 assembly language should have no trouble creating assembly DLL files for use in visual basic. The process of creating an assembly DLL file is the same as creating a DLL file in C++, and the calling convention is still standard call; however, programmers need to know some information about variable sizes and structure before they can create assembly DLL files for visual basic.
The following information is how variables correspond between visual basic and assembly language. This list is not complete, but other variables can be looked up on MSDN.
Visual basic – Assembly
byte – BYTE
Char – BYTE
boolean – WORD
Integer – WORD
Long – DWORD
Short – DWORD
String – DWORD Variable is passed by pointer.
While variables correspond quite harmoniously between visual basic and assembly, arrays are a different story. Visual basic does not store arrays in a straight forward manner; instead, visual basic stores arrays in an OLE_SAFEARRAY structure. When programmers wish to pass arrays to assembly language DLL files, they must work with the structure of the array.
SAFEARRAYBOUND struct
cElements DWORD ? ;Number of Elements
lLbound DWORD ? ; Lower Boundary
SAFEARRAYBOUND ends
OLE_SAFEARRAY struct
cDims WORD ? ; Number of dimensions
fFeatures WORD ? ; Bitfield indicating attributes
cbElements DWORD ? ; size of an element of the array
cLocks DWORD ? ; lock counter 0=Locked
pvData DWORD ? ; Pointer to data
rgsabound SAFEARRAYBOUND <> ; Contains info for dimensions
OLE_SAFEARRAY ends
Finally, here the following is an example of how to reference the structure from MASM32.
testfunction proc myArray:DWORD
mov eax, myArray
mov edx, [eax] ;EDX now has our safearray.
'Assign 1st value to eax.
mov eax, (OLE_SAFEARRAY ptr [edx]).pvData
ret
testfunction endp
Read More......